Essential Java Best Practices for Developers In 2023
Java is one of the widely used programming languages for developing applications. Scalable, security, faster performance, and flexibility are features of Java. Java can be used in various varieties of the application. In Java, a single operation can be performed in many ways. So it is essential to follow Java practices and Java coding practices while developing Java applications.
By following the Java coding standard, developers can avoid common issues as these Java coding standards are there because these are the best ways to implement something specific. That can avoid bugs, issues, memory leaks, and performance issues. Below are the coding standards in Java to improve your readability and the development speed of your applications.
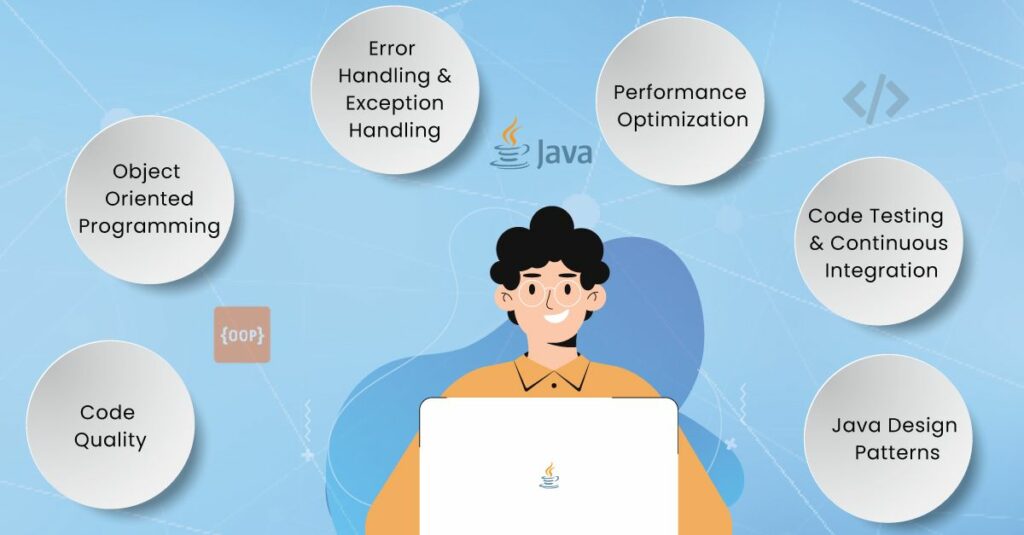
The importance of Java best practices
Java best practices are crucial for writing efficient, maintainable, scalable code. They enhance code readability, reduce bugs, improve performance, and facilitate collaboration. Adhering to best practices ensures code quality, promotes reusability, and leads to more robust and reliable Java applications.
Code Quality and Maintainability
Good code quality and durability are paramount while developing any application software. You can follow these Java coding best practices to make it more efficient.
Writing clean and readable code
Writing clean and readable code in Java is crucial for maintainability and collaboration. It enhances code comprehension and reduces potential bugs, making it easier to debug and modify in the future.
Commenting and documentation
Commenting and maintaining documentation in your code can help other developers to understand existing functionality. It also reduces the initial time of onboarding new developers on the project. We can do inline commenting on methods, classes, and config files. You can also find libraries that support documentation, like OpenAPI.
Consistent naming conventions
In every programming language, you need to understand their naming conventions. For specific to Java, we use different naming conventions for different things, such as for variables, we use camelcase. For constants, we use strict uppercase only. This way, we can understand its functionality and use by looking at names.
Object-Oriented Programming Principles
OOP’s principles guide the design and implementation of object-oriented software systems. They help developers create modular, reusable, and maintainable code.
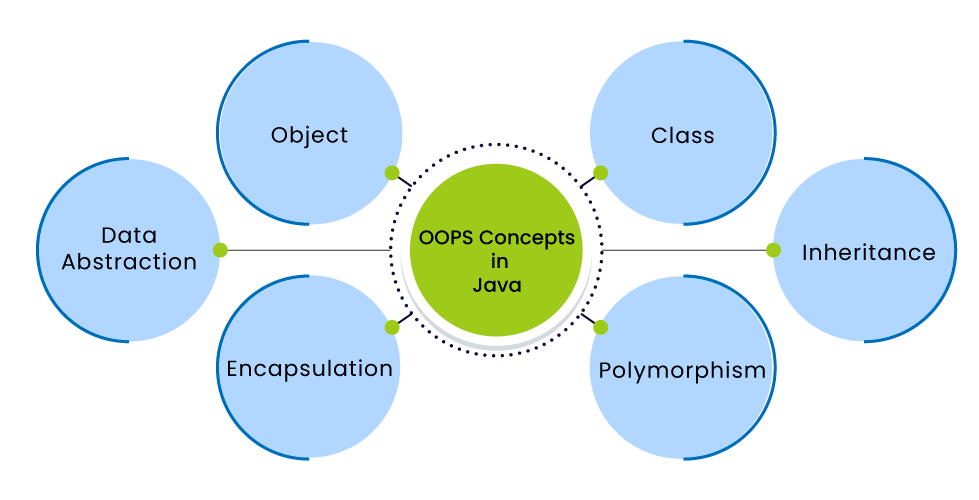
There are four primary OOP principles which are listed below:
Encapsulation
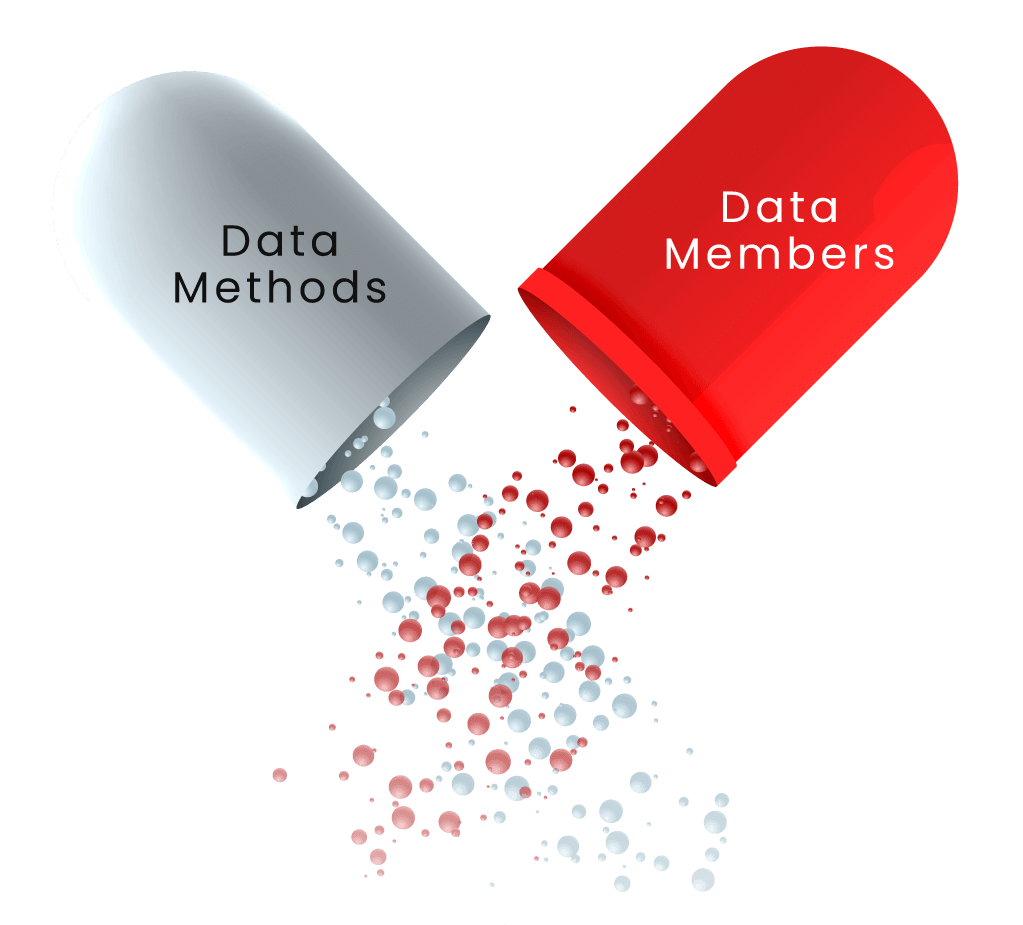
Encapsulation is a fundamental principle of OOPs that is important in designing robust and maintainable Java code. It is about wrapping data and related methods into a single unit.
Inheritance
In Java programming, inheritance plays a vital role in code reuse and organization. Developers can extend functionality by creating subclasses that inherit attributes and behaviors from superclasses. There are different types of inheritance:
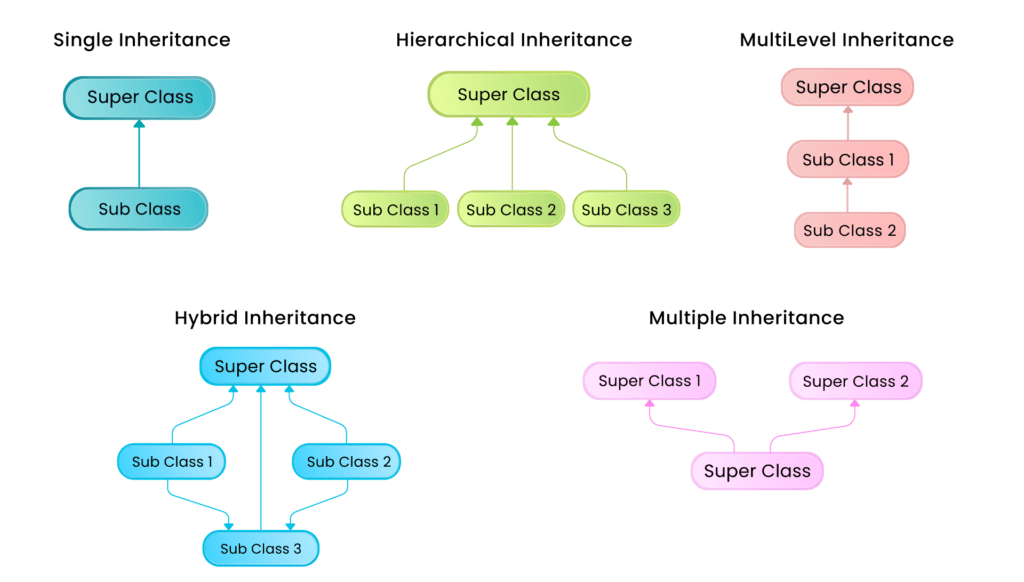
1. Single Inheritance : A class can inherit from a single superclass, forming a linear hierarchy.
2. Multiple Inheritance : Java supports multiple inheritances through interfaces. A class can implement multiple interfaces, inheriting their method signatures while providing its implementations.
3. Hierarchical Inheritance : Multiple classes inherit from a single superclass, allowing code reuse among related classes.
4. Hybrid Inheritance : Hybrid inheritance combines single inheritance, multiple inheritances through interfaces, and multilevel inheritance to create complex class hierarchies.
5. These inheritance types enhance code reuse and extend the functionality of parent classes.
Polymorphism
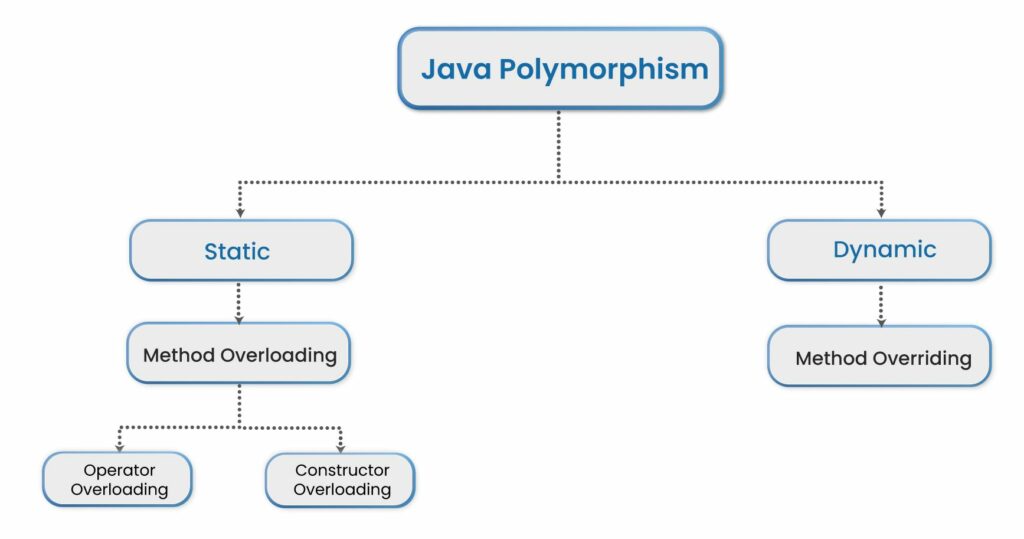
Polymorphism is an essential characteristic of Java that enables objects to take multiple forms. This encourages flexibility and code reuse.
In Java, polymorphism is achieved through method overriding and method overloading. Method overriding allows subclasses to provide their implementations of methods inherited from superclasses.
Method overloading enables the creation of multiple methods within a class with the same name but different parameters.
Abstraction
Abstraction allows developers to focus on essential functionality by simplifying complex real-world entities. Abstract classes and interfaces are used to achieve abstraction. Abstract classes are blueprints for subclasses, while interfaces define contract requirements for classes to adhere to.
Error Handling and Exception Management
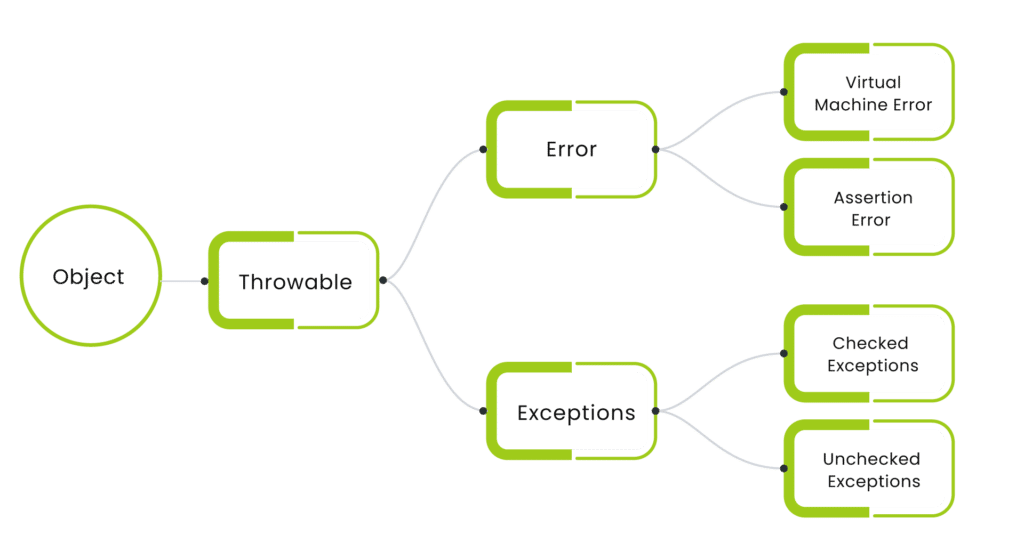
Java provides various tools and mechanisms for handling errors and exceptions.
Some of them are listed below.
Using try-catch blocks effectively
Effective use of try-catch blocks can prevent program crashes and improve code reliability. It creates more stable and maintainable software systems. Excessive or poorly designed exception handling can add unnecessary complexity and slow system performance.
Creating custom exceptions
Creating custom exceptions is a powerful technique for handling specific error scenarios.
This approach enables developers to communicate and handle exceptions more meaningfully and precisely. Custom exceptions make adding custom fields, methods, and behaviors possible, resulting in more robust and maintainable Java code.
These exceptions can be designed to handle unique business logic errors. They can also handle input validation failures or other exceptional conditions specific to the application’s domain.
Handling checked and unchecked exceptions
Exceptions are classified into two types. Checked and unchecked. A checked exception is an exception checked by the compiler during compilation.
Using the throws keyword, it should be caught or declared within a method signature. To handle checked exceptions in Java, developers must use try-catch blocks or declare them in method signatures.
The compiler excludes checking unchecked exceptions, allowing them to be caught or left unhandled. You can also use try-catch blocks for unchecked exceptions and leave them unhandled if desired.
Performance Optimization
Performance optimization is aimed at improving the efficiency and speed of software applications. To achieve optimal performance, developers employ various strategies, including efficient use of data structures and optimal memory management.
Efficient use of data structures
The efficient use of data structures plays an essential role in performance improvement. Choosing suitable data structures is vital for optimizing performance.
Commonly used options include arrays, lists, and maps. You should select each data structure based on the specific needs of your application. Making an appropriate choice can have a significant impact on the efficiency of your program.
Developers can minimize the time complexity of their algorithms by choosing suitable data structures. Efficient search, insert, and delete operations are vital to achieving this goal. By optimizing these operations, overall performance can be significantly improved.
Optimal memory management
Optimal memory management is another essential factor in optimizing performance. Java’s garbage collection mechanism automatically released memory occupied by unused objects.
However, poor memory management can cause excessive pauses in garbage collection, which can adversely affect performance. Developers can minimize object creation, reuse, and optimize memory usage by explicitly turning off unused references and allocating and deallocating memory efficiently.
Minimizing synchronization overhead
Minimizing synchronization overhead is essential when programming concurrently. Mechanisms such as locks and synchronized blocks ensure thread safety but incur overhead.
By minimizing the use of locks, developers can reduce blockers between threads. Synchronizing only critical sections of code is another way to improve performance. Using non-blocking algorithms and data structures like Java.util.concurrent package can also improve performance in concurrent scenarios.
Java Concurrency Best Practices
Concurrency is integral to modern software development, and Java provides powerful tools and utilities for managing concurrent operations. However, following the Java coding best practices checklist is vital to ensure thread safety, avoid deadlocks, and manage race conditions effectively.
Thread safety
Thread safety is paramount in concurrent programming. It involves designing code so multiple threads can safely access shared resources without conflicts or unexpected behavior.
Mechanisms should be used to protect critical sections of code. These mechanisms include locks, synchronized blocks, and concurrent data structures. Its use can prevent data corruption.
Using Java concurrency utilities
Java provides an extensive set of concurrency, such as Java.util.concurrent package. It provides thread-safe collections, classes, and an execution framework.
These utilities simplify concurrent programming. They use efficient thread management and its techniques. This reduces the risk of errors and improves performance.
Properly handling deadlocks and race conditions.
Deadlocks and race conditions are common pitfalls in concurrent programming. A deadlock occurs when two or more threads are waiting for resources. These resources are occupied by each other, and neither thread can continue. Acquiring locks in a consistent order and releasing locks accordingly is essential to prevent deadlocks.
Java Design Patterns
Java design patterns are reusable solutions to common software design problems. They provide a structured approach to developing flexible and maintainable code. Four common design patterns in Java are the Singleton, Factory, Observer, and Strategy.
Singleton pattern
The singleton pattern ensures that only one class instance exists for the application. This is useful when you need a single global access point, such as for loggers or database connections.
Factory pattern
The Factory pattern provides a way to create objects without specifying a concrete class. It encapsulates object creation logic, promotes loose coupling, and enhances code extendibility.
Observer pattern
The Observer pattern establishes a one-to-many relationship between objects. When an object’s state changes, all objects that depend on it, called observers, are automatically notified and updated. This pattern facilitates isolation and allows efficient communication between objects.
Strategy pattern
Strategy patterns allow you to choose algorithms or behaviors at runtime. Encapsulate replaceable algorithms in a separate class so they can be easily interchanged at runtime. This promotes code reuse, flexibility, and ease of maintenance.
Code Testing and Continuous Integration
Code testing and continuous integration are integral components of software development that ensure codebases’ quality, reliability, and stability. These Java practices help identify and fix issues early in development, improving software performance.
Unit testing with JUnit
Unit testing, enabled by frameworks like JUnit in Java, is fundamental to code testing. It involves writing and executing test cases to validate individual code units, such as methods or functions. By testing units in isolation, developers can detect and fix bugs more efficiently.
Integration testing
The primary objective of integration testing is to test how different components of a system interact with each other. It ensures that various modules and subsystems work seamlessly when combined. Integration testing verifies the proper communication and data flow between these components, providing confidence in the system’s functionality.
Leveraging continuous integration tools
Developers can catch defects early by leveraging unit testing. They can also reduce manual effort through integration testing. Additionally, developers can improve the software development by utilizing continuous integration tools. These Java practices promote code stability and maintainability.
Java Security Best Practices
Java security best practices are essential for developing secure and robust applications. By adhering to these practices, developers can protect their applications from potential risks and ensure data integrity and privacy.
Secure coding practices
Adopting secure Java coding standard practices is an essential element of Java security. This involves following Java coding guidelines and these Java coding standards that mitigate common security risks.
It includes practices like avoiding the use of deprecated or insecure APIs. Properly handling exceptions is another vital practice. Enforcing strong authentication and authorization mechanisms is also necessary.
Input validation and output sanitization
Input validation and output sanitization are vital steps in preventing security breaches. Validating user input is crucial in ensuring that only expected data is processed. It helps to reduce the risk of injection attacks, such as SQL injection or cross-site scripting (XSS). By validating input, developers can prevent malicious code from being executed and ensure the security and integrity of their applications.
Handling sensitive data
Handling sensitive data securely is another crucial aspect of Java security. This involves appropriately encrypting sensitive information, such as passwords or credit card details, during transmission and storage. Securely managing secrets, such as API or cryptographic keys, is critical to prevent unauthorized access.
Conclusion
In conclusion, adopting Java coding standards and Java best practices are essential for developing high-quality and secure software. Developers can minimize the risk of security risks by implementing secure coding practices. They can ensure the reliability of their code through input validation, output sanitization, and handling of sensitive data. Following Java coding standards and best practices is crucial for building robust and trustworthy software applications. For more information, you can contact plenty of other best Java coding practice sites.
Unlock the potential of your software solutions with Thinkitive’s exceptional Java development services experience excellence by hiring Java programmers through our free trial. Get started now!
Adopting Java best practices for higher-quality software development
As a Tech lead, it’s highly recommended to convey best practices to implement while building software in your team. Writing clean code and entirely using language features lead to easy debugging and writing test cases. Even most of the pro developers fail to resolve bugs if the code is not well structured and end up re-writing the code. Adopting good practices may take some time, but it will cost you less later with time and money.
Frequently Asked Questions
Best practices offer improved code quality, leading to cleaner, more maintainable code with reduced complexity and fewer bugs. Consistent practices can enhance code readability, making it easier for developers to understand and debug the codebase.
You can always stay up-to-date by following the Oracle portal, which officially maintains Java. Also, there are many sites you can find about best practices.
- Checkstyle :Helps enforce coding conventions and formatting standards in Java code.
- PMD : Identifies potential issues and suggests improvements in Java code, including style violations and common programming mistakes.
- FindBugs :Scans Java bytecode to detect common bugs, coding errors, and performance issues.
- SonarQube : Offers comprehensive code quality analysis, highlighting code smells and security vulnerabilities and enforcing best practices.
- IntelliJ IDEA and Eclipse :Popular IDEs with built-in code analysis features that detect coding standards violations and provide feedback within the code editor.
- These tools aid in maintaining code quality, improving readability, and ensuring adherence to Coding best practices.
Hey There. I discovered your weblog the use of msn. This is an extremely well written article.
I’ll make sure to bookmark it and return to learn extra of
your helpful info. Thank you for the post. I’ll definitely comeback.
Keep on working, great job!
Greetings! Very helpful advice within this article!
It is the little changes that make the most important changes.
Thanks a lot for sharing!