Top 5 ReactJS Best Practices and Tips to Follow In 2023
React is a popular JavaScript library for building user interfaces, and it has gained even more traction in recent years due to its versatility and ability to be used for web and mobile applications.
However, as a React developer, understanding how the library works is one of many things you need to build user-friendly, easily scalable, and maintainable projects. It’s also necessary to understand certain conventions and React best practices to enable you to write clean React code.
This will help you serve your users better and make it easier for you and other developers working on the project to maintain the code base. When working with React in 2023, here are some best practices to remember.
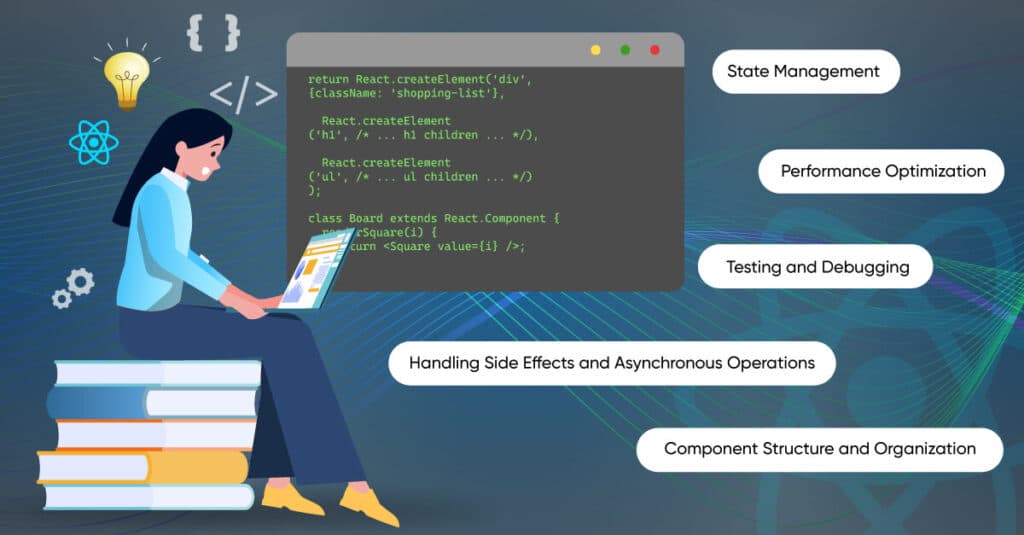
1. Component Structure and Organization
Within a React app, a component-centric folder structure will help maintain organization. One folder stores all the files regarding one component.
Create a Navbar folder, then place the component file, style sheets, and other required JavaScript/asset files inside it. In addition, debugging, sharing, and reusing components become more accessible with a folder that stores everything. Opening that folder then will give you a clear view of the element’s operation.
Following are other react best practices :
Generally, index files can help abstract the implementation specifics of a component file. For the Navbar example, create a folder named Navbar, and within this folder, create an index.js (or .ts) file. Reusable components live in their folder. To keep them organized, components used by various application sections can be stored in a folder dubbed components. With this, finding them will be easy.
Utility functions should be stored in a separate folder like lib or helpers. The goal is to make it easier to manage and reuse them later on.
2. State Management
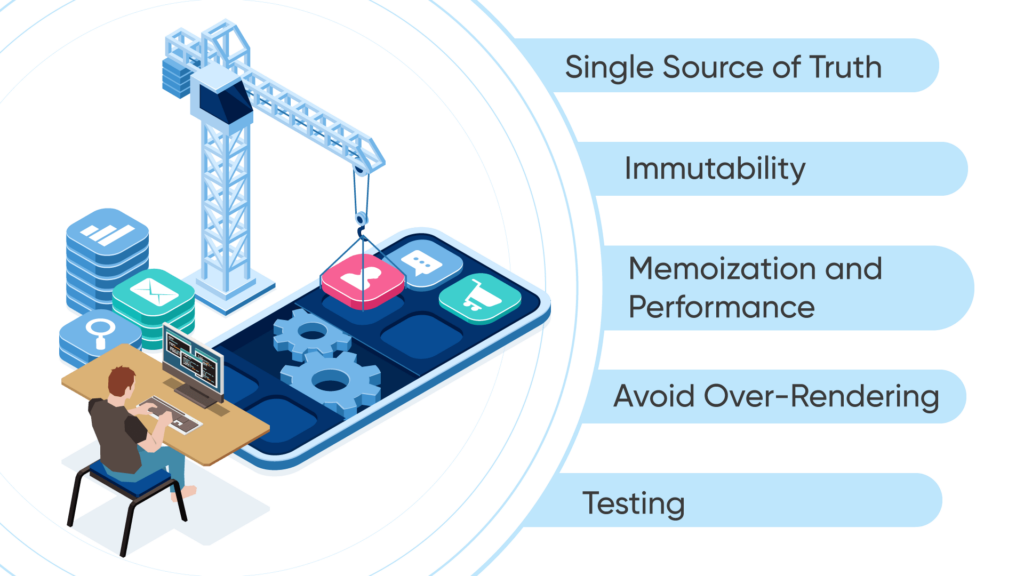
Building React apps requires effective state management strategies. Many developers prefer these two popular methods when choosing between Redux, Context, and other alternatives. With context, you may share the state amongst parts of your application. Depending on individual needs, both choices offer advantages and disadvantages.
Redux and similar libraries offer much-needed support when managing complex app states for more prominent apps.
Beyond Redux lie other choices like Recoil, MobX, and Zustand. When dealing with multi-level component architecture, rely on the power of Context API.
State management tips for ReactJS include optimizing performance through reduced re-renders upon change.-
Single Source of Truth:
One spot should store all the pieces. Data duplication and inconsistency prevention are at play here.
Immutability:
Never mutate state directly. Making changes requires a fresh creation of the state. Through this process, unexpected results can be avoided by monitoring changes closely.
Memoization and Performance:
Memoization strategies like React. Memo and the useMemo hook help avoid unnecessary re-rendering.
Avoid Over-Rendering:
Updates should only occur if necessary; caution against excessive modification of components. These techniques will aid in improving rendering by optimizing them.
Testing:
To confirm state management operates effectively, write tests. Testing React components and states become more straightforward with tools such as Jest and React Testing Library.
3. Handling Side Effects and Asynchronous Operations
Handling side effects and asynchronous operations is a common challenge in React applications. Below are some of the common react best practices that you can follow to handle side effects and asynchronous operations –
Use useeffect for Side Effects:
The useEffect hook is the primary tool for handling side effects in functional components. It allows you to perform actions after rendering, such as data fetching, subscriptions, and DOM manipulation.
Fetch Data with useEffect:
Fetch data from APIs or external sources inside the useEffect hook. This ensures that data fetching occurs after the component has been rendered.
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(‘api_url’);
const data = await response.json();
// Update state with fetched data
} catch (error) {
// Handle error
}
};
fetchData();
}, []);
Dependency Arrays in useEffect:
Specify dependencies in the array passed as the second argument to useEffect. This helps control when the effect runs.
useEffect(() => {
// Effect logic
}, [dependency1, dependency2]);
Cleanup in useEffect:
Return a cleanup function from the useEffect callback to handle cleanup, such as unsubscribing from subscriptions or clearing timers.
useEffect(() => {
const timerId = setInterval(() => {
// Timer logic
}, 1000);
return () => {
clearInterval(timerId); // Cleanup
};
}, []);
Avoid Fetching in Every Render:
Use conditional checks within useEffect to avoid unnecessary data fetching on every render.
Async/Await in useEffect:
You can use async/await syntax inside useEffect for cleaner code when dealing with asynchronous operations.
Redux Thunk or Saga:
If you’re using Redux, consider middleware like Redux Thunk or Redux Saga to manage asynchronous actions and side effects within Redux.
Promises and Async Functions:
Use promises or async functions to handle multiple asynchronous operations sequentially or in parallel.
Error Handling:
Implement error handling for asynchronous operations, such as network errors during data fetching. Use try/catch blocks or promise catch methods.
Avoid setState in Unmounted Components:
Check if a component is still mounted before performing actions that could lead to state updates in unmounted components.
Remember that maintaining a clear separation between UI rendering and side effects is essential for code readability and maintainability. By following these React best practices, you can effectively manage your React applications’ side effects and asynchronous operations.
4. Performance Optimization
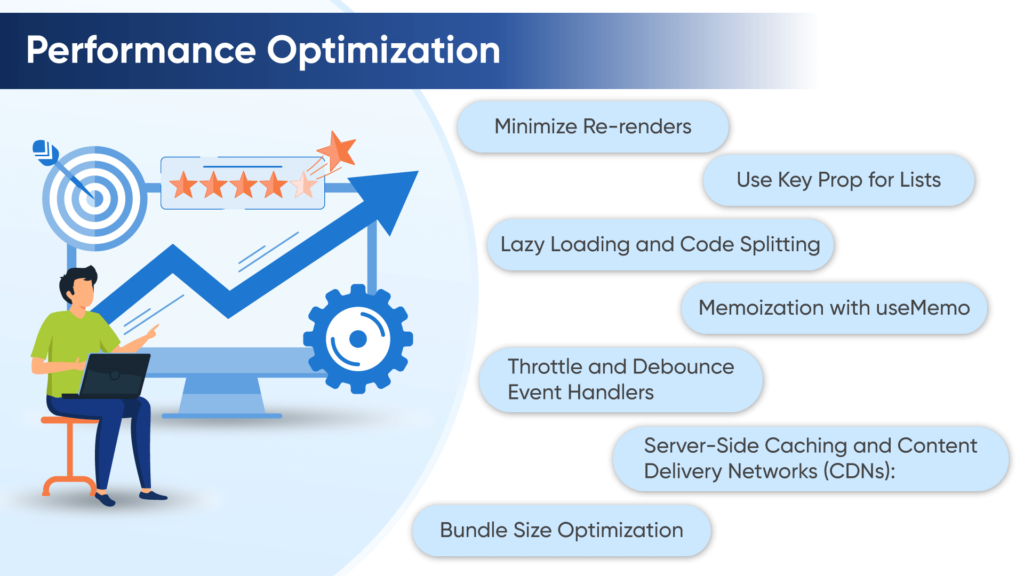
Performance optimization is crucial for delivering fast and efficient React applications. Here are some best practices in Reactjs to consider for optimizing the performance of your applications:
Minimize Re-renders:
Components must be optimized so they only re-render when necessary. Unwanted re-renders can be avoided thanks to techniques like React. memo, PureComponent, and shouldComponentUpdate.
Use Key Prop for Lists:
Ensuring each item has an exclusive identifier through the map function is essential. Through this efficiency feature, React updates and renders lists quickly.
Lazy Loading and Code Splitting:
You can successfully implement code splitting and lazy loading with React.lazy and dynamic import(). Only load what you need now based on your current route or view.
Memoization with useMemo:
Using the useMemo hook helps avoid repetitive recalcs by storing pricey calculations.
Throttle and Debounce Event Handlers:
Debounding or throttling event handlers help regulate how frequently actions like scrolling and inputs occur.
Server-Side Caching and Content Delivery Networks (CDNs):
With caching on the server side and CDNs, our load times will be faster and less taxing on servers.
Bundle Size Optimization:
Efficiently bundle your code using tools such as Webpack and Babel, thereby minimizing the size of your JavaScript bundles. Avoid including unnecessary dependencies.
5. Testing and Debugging
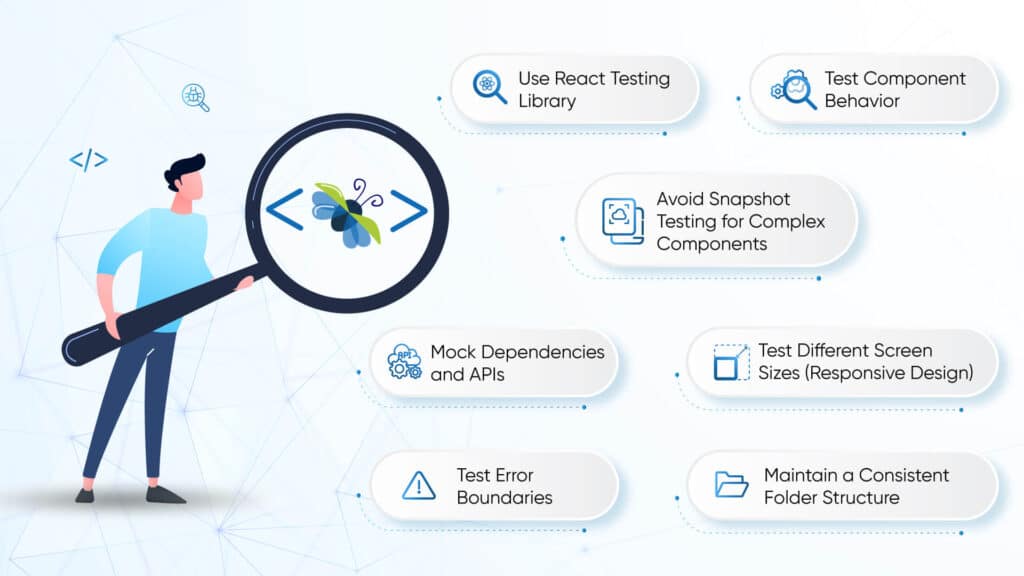
By writing component tests, errors can be minimized when coding React. Adequate testing ensures developers can expect parts to behave accordingly and reduces issues when implementing React.
Success depends on how well the freshly integrated codes mesh with the existing ones within a project. Existing functionality may break, chance upon. By giving coding examinations to every person, the problem will be resolved. Within the directory, create a test directory to conduct relevant tests.
Divide tests in React into testing the functionality of components and tests on the application once it renders in the browser. Use cross-browser testing tools that can help with the objective of testing the latter category. For the former, use a JavaScript test runner, Jest, to help emulate the HTML DOM using jsdom to test React components.
An utterly accurate test is only possible in a browser long associated with the device. Also, Jest provides a good approximation of the actual testing environment that can significantly assist a developer in the project’s development phase. Also, this principle serves as React practical advice for improving code quality.
Writing practical unit tests for React components is essential to ensure their functionality, stability, and maintainability. Here are some React js practices for writing unit tests:
Use React Testing Library:
Use React Testing Library (RTL) for testing React components. It encourages testing the component’s behavior from a user’s perspective.
Test Component Behavior:
Focus on testing user interactions, such as clicking buttons, typing into inputs, and submitting forms.
Avoid Snapshot Testing for Complex Components:
While snapshot testing can be helpful, avoid relying solely on it for complex components with dynamic content. It can lead to brittle tests that break on minor changes.
Mock Dependencies and APIs:
Mock external dependencies and APIs using Jest’s jest.mock() or other mocking libraries. This isolates your component from external behavior.
Test Different Screen Sizes (Responsive Design):
You should test your component’s responsiveness by changing the viewport size and checking how the component behaves.
Test Error Boundaries:
If your component uses error boundaries, test that they catch and handle errors as expected.
Maintain a Consistent Folder Structure:
Organize your test files in a structure that mirrors your component structure. This makes it easier to locate and manage tests.
Debugging tools and browser extensions are essential for identifying and fixing issues in your web applications. Here are some Reactjs tips for using debugging tools and browser extensions effectively:
Browser Developer Tools:
Inspect Elements:
Use the “Inspect” tool to examine and modify the HTML and CSS of elements. Hover over elements in the Elements panel to highlight them on the page.
Performance Profiling:
Use the Performance tab to profile your application’s performance. Identify bottlenecks, excessive reflows, and long tasks affecting the user experience.
Memory Profiling:
Use the Memory tab to profile memory usage and identify memory leaks. Take heap snapshots to analyze memory allocation and deallocation.
Sources / Debugger:
Set breakpoints in your JavaScript code to pause execution and inspect variables. Step through code execution using the “Step Over,” “Step Into,” and “Step Out” buttons.
Responsive Design Mode:
Test your application’s responsiveness using the responsive design mode to simulate various device sizes.
Browser Extensions:
React Developer Tools Extension:
Install the React DevTools extension for your browser to enhance your ability to inspect and debug React applications.
Redux DevTools Extension:
If you’re using Redux, the Redux DevTools extension helps you monitor Redux actions, state changes, and time-travel debugging.
CSS Browser Extensions:
Extensions like “Web Developer” or “CSS Viewer” allow you to inspect and manipulate CSS on any web page.
API Testing Extensions:
Tools like “Postman” or “RESTClient” help you test APIs by sending requests and receiving responses directly within your browser.
Final Thoughts
To conclude, learning to use React is optional to create outstanding web apps. As with any other framework like Angular, Vue, and so on, there are best practices that you should follow to help you build efficient products.
Following these Reactjs tips and tricks helps your app and has advantages for you as a frontend developer — you learn how to write efficient, scalable, and maintainable code and stand out as a professional in your field.
So, when building your next web app with React, bear these best practices in mind to make using and managing the product easy for your users and developers.
Frequently Asked Questions
To optimize a React project, use code splitting, minifying assets, lazy loading, CDNs, and implementing SSR or SSG. Profile performance, optimize images, reduce third-party dependencies, and leverage memoization. Employ PureComponent/React.memo, virtualization, and manage state efficiently. Test on various devices, browsers, and networks while staying updated with best practices.
While it may be true, in 2023, React will be a great web development framework. By 2021, ReactJS had surpassed jQuery as the most popular web framework programmers use worldwide. It’s simple to learn and has many advantages over other JavaScript libraries, including more well-known ones.
The “best” architecture for React depends on the project’s complexity and needs. However, a popular choice is the “component-based” architecture, where the app is divided into reusable components that manage their state and UI. Combine this with state management tools like Redux or React Context for the global state and follow patterns like Container/Presentational components to separate concerns. Strive for a clear data flow and maintainable structure while adapting to project requirements.
React’s performance is enhanced through virtual DOM, which minimizes actual DOM updates, and reconciliation algorithms that intelligently update only changed components. Code splitting reduces initial load times, while React’s built-in memoization and PureComponent optimize rendering. Efficient state management, component hierarchy, and browser caching contribute to faster user experiences.
Profiling tools help identify bottlenecks, while server-side rendering and optimized images enhance performance. Thus, continuously applying these strategies maintains React’s speed and responsiveness.
Hi there! I’m at work surfing around your blog from my new apple iphone!
Just wanted to say I love reading through your blog and look forward to all your posts!
Carry on the excellent work!